햄버거 하우스 게임
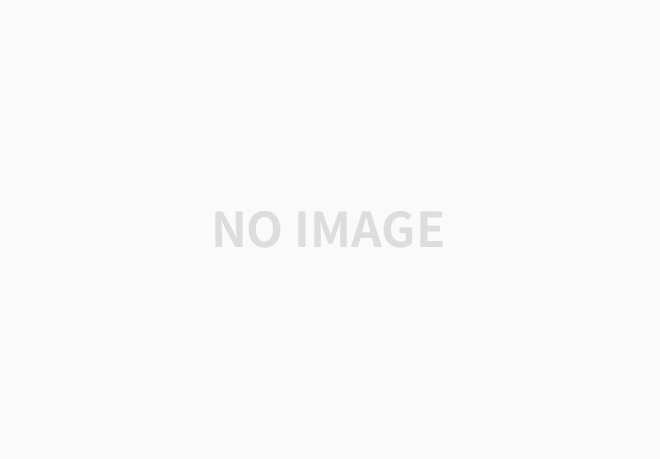
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Edu+VIC+WA+NT+Beginner:wght@700&display=swap" rel="stylesheet">
<title>day04_ex01.html</title>
<style>
* {}
body {
/*background-image:url(img/햄버거.jpg);
background-repeat:repeat-x ;*/
background-color: black;
background-image: url(img/햄버거게임.PNG);
background-size: cover;
background-repeat: no-repeat;
background-position: center;
}
h1 {
text-align: center;
color: rgb(255, 34, 34);
font-family: 'Edu VIC WA NT Beginner', cursive;
}
div.main {
margin-left: 300px;
}
ul.imgGrp {
border: 1px solid rgb(0, 0, 0);
padding: 0;
margin: 0;
width: 120px;
float: left;
}
ul.imgGrp > li {
border: 1px solid black;
width: 120px;
height: 100px;
box-sizing: border-box;
text-align: center;
line-height: 100px;
list-style: none;
}
#box {
border: 3px solid black;
height: 100px;
overflow: hidden;
width: 600px;
margin-left: auto;
margin-right: auto;
margin-top: 100px;
}
</style>
</head>
<body>
<h1>💥햄버거 하우스 게임💥</h1>
<hr>
<!--
<div class="main"><img src="img/햄버거.jpg" alt=""></div>
-->
<div id="box"></div>
<script>
function ImgGrp(parentElement) {
this.parentElement = parentElement;
this.ulTag = null;
this.init();
}
ImgGrp.prototype.init = function() {
console.log('init ...');
//console.log(this.parentElement);
//ul태그를 만들어서 parentElement에 append 한다.
this.ulTag = document.createElement('ul');
this.ulTag.className = 'imgGrp';
this.ulTag.style.position = "relative";
var colos = ['red','green','blue','orange','brown'];
for(var i=0; i<10; i++){
this.liTag = document.createElement('li');
this.liTag.innerText = i+1;
this.liTag.style.backgroundColor = colos[i%colos.length];
this.ulTag.appendChild(this.liTag);
}
this.parentElement.appendChild(this.ulTag);
};
ImgGrp.prototype.start = function() {
console.log('Start!');
var x = 0;
var target = (Math.floor(Math.random()*10000 / 100)) * 100;
var ul_tag = this.ulTag;
//this.setInterval = window.setInterval;
var interval = setInterval(function() {
//여기서 this는 window가 된다.
x -= 30;
console.log(x);
ul_tag.style.top = x + "px";
if(x <= -(target%900) ) {
clearInterval(interval);
ul_tag.style.top = -(target%900) + "px";
}
}, 1000/30);
};
window.onload = function() {
var box = document.getElementById("box");
//console.log(box);
var imgGrp01 = new ImgGrp(box);
imgGrp01.start();
var imgGrp02 = new ImgGrp(box);
imgGrp02.start();
var imgGrp03 = new ImgGrp(box);
imgGrp03.start();
var imgGrp04 = new ImgGrp(box);
imgGrp04.start();
}
</script>
</body>
</html>
퍼즐 게임
이건 문제가 있다. A A 가 선택시 노출이되지만, A와 다른 문자를 선택시 고정되어야하는데 함께 사라진다.
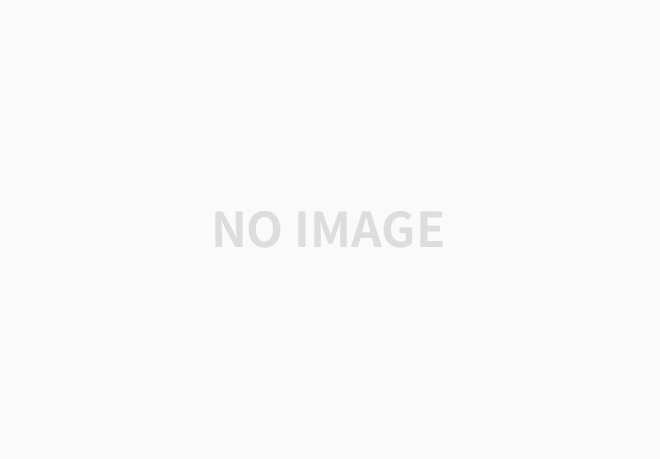
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>민트가 좋아 퍼즐</title>
<style>
*{
background-color: #99f2c8;
}
div#puzzle {
border: 3px solid black;
width: 400px;
height: 400px;
padding: 0;
}
#puzzle div.cell {
width: 100px;
height: 100px;
border: 5px solid rgb(0, 0, 0);
box-sizing : border-box;
float: left;
margin: 0;
text-align: center;
line-height: 100px;
background: #1f4037; /* fallback for old browsers */
background: -webkit-linear-gradient(to top, #99f2c8, #1f4037); /* Chrome 10-25, Safari 5.1-6 */
background: linear-gradient(to top, #99f2c8, #1f4037); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
}
</style>
<script>
class PuzzleGame {
constructor() {
this.puzzle = null;
this.cells = [];
this.init();
this.firstCell = null; //추가
}
init() {
const body = document.body;
body.innerHTML = `<h1>MINT Matching Puzzle</h1><hr />`;
this.puzzle = document.createElement("div");
this.puzzle.id = "puzzle";
body.appendChild(this.puzzle);
for(var i=0; i<16; i++) {
var cell = document.createElement("div");
cell.className = "cell";
cell.innerText = i;
this.puzzle.appendChild(cell);
this.cells.push(cell);
}
this.cells.forEach(function(item, i) {
let charCode = i%8+65;
item.innerHTML = String.fromCharCode(charCode);
});
}
swap(i,j){
let tmpTxt = this.cells[i].innerText;
this.cells[i].innerText = this.cells[j].innerText;
this.cells[j].innerText = tmpTxt;
}
//아래 compare ()추가
compare(cell) {
//첫 번째 클릭 cell.ch와 두 번째 클릭 cell.ch와 비교
//같으면 남기고 다르면 숨기기
// console.log(cell.ch);
if (this.firstCell == null) {
this.firstCell = cell;
this.firstCell.innerHTML = cell.ch;
} else {
if (this.firstCell.ch == cell.ch) {
cell.innerHTML = cell.ch;
this.firstCell = null;
}
else {
cell.innerHTML = cell.ch;
setTimeout(() => {
cell.innerHTML = ""; // cell 을 빈칸으로
this.firstCell.innerHTML = ""; //firstcell을 빈칸으로
this.firstCell = null; //firstcell 을 null 값으로
}, 1000); // 1초
}
}
}
start(){
for(let i=0; i<50; i++){
let idx1 = Math.abs(Math.floor(Math.random()*16));
let idx2 = Math.abs(Math.floor(Math.random()*16));
this.swap(idx1, idx2);
}
setTimeout(()=>{
//모든 요소 숨기기
this.cells.forEach((item,i)=>{
item["ch"] = item.innerText;
item.innerHTML="";
item.addEventListener('click',(e)=>{
e.currentTarget.style.backgroundColor = "red"; //추가
this.compare(e.currentTarget);
if (this.checkerCounter == 8) { //추가
this.cells.forEach((item, i) => {//추가
item.style.backgroundColor = "green";//추가
item.style.color = "white";//추가
});
}
})
});
},2000);
}
}
function main() {
new PuzzleGame().start();
}
window.addEventListener("load", main);
</script>
</head>
<body>
</body>
</html>
'웹 개발자 준비 과정🐳' 카테고리의 다른 글
day09: 학교 홈페이지(전자칠판,채팅) 만들기😄 (3) | 2022.07.31 |
---|---|
day08: 자바스크립트를 이용하여 snakegame 만들기😬 (1) | 2022.07.31 |
day06: 드래그 앤 드롭(drag and drop)😮💨 (0) | 2022.07.26 |
day05: 개인 홈페이지 만들기_서울소개(html,css,js,bootstrap,firebase)🤩 (0) | 2022.07.26 |
day04: 부트스트랩_jeju (html,css,js,bootstrap)😀 (0) | 2022.07.26 |